1. 基本指令(编译、测试、部署)
1.1 执行 npx hardhat
报错
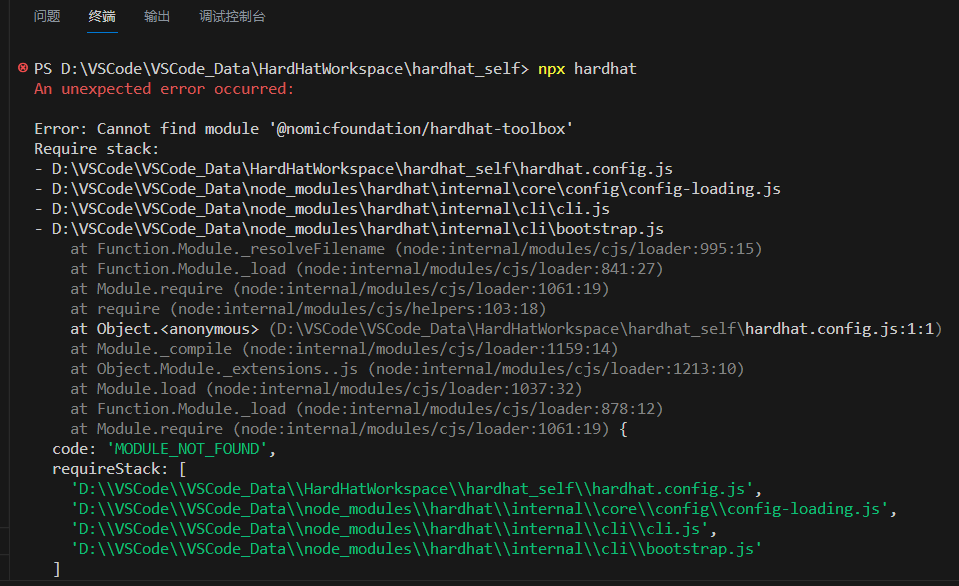
原因:
缺少”@nomicfoundation/hardhat-toolbox
“Hardhat插件。
解决方案:
1
| npm install --save-dev @nomicfoundation/hardhat-toolbox
|
如果报以下错误,则再执行一遍 npm install --save-dev @nomicfoundation/hardhat-toolbox
即可
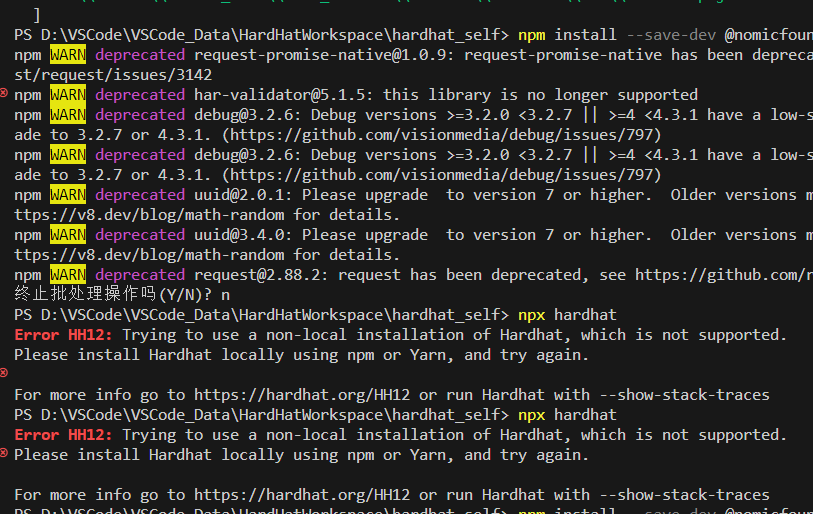
1.2 编译合约

1.3 运行测试
1
| npx hardhat test test/Lock.js
|
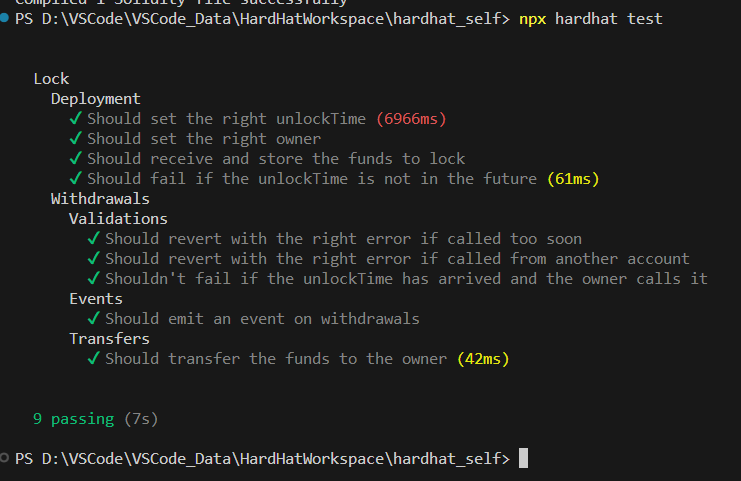
如果因为之前在对合约的创建的时候,文件名和合约名不一样,重命名会出现test
报错的情况,解决方法就是,将当前工作目录下编译生成的artifacts
和cache
文件删除,再执行npx hardhat compile
,重新编译。
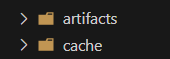
1.4 运行js文件(部署合约)
1
| npx hardhat run scripts/deploy.js
|

1.5 将钱包或dapp连接到hardhat网络
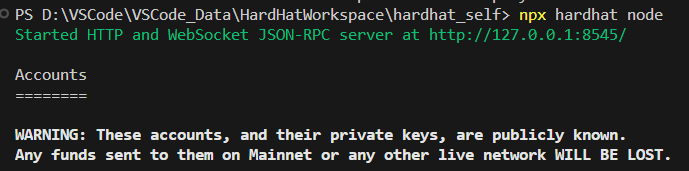
1
| npx hardhat run scripts/deploy.js --network localhost
|
注:需要将本地网络启动,即需要开启两个终端,一个用于挂载网络,一个用于测试

2. 创建一个基本的项目
2.1 创建命令
在你要创建项目的文件夹(空文件夹)下执行如下命令:
初始化的项目结构如下:
1 2 3 4
| contracts/ scripts/ test/ hardhat.config.js
|
contracts/
是合同源文件所在的位置。
test/
是你的测试应该去的地方。
scripts/
这是简单的自动化脚本所在的位置。
3. 对合约进行测试
3.1 语法格式
1 2 3 4 5 6
| const { ethers } = require("hardhat"); describe("",function(){ it("", async function () { }); });
|
3.2 测试合约(无参构造器)
MyContract1.sol
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| // SPDX-License-Identifier: UNLICENSED pragma solidity ^0.8.0;
import "hardhat/console.sol";
contract MyContract1 { address owner;
constructor() { owner = msg.sender; }
function getOwner() external view returns(address) { console.log(unicode"这是一个测试合约!"); return owner; }
}
|
解读:
import "hardhat/console.sol"
:其作用是从hardhat
文件夹下引入console.sol
合约 (但是我翻了个遍,在本地都找不到这个合约);
console.log()
:其作用是在控制台打印输出自定义结果;
unicode"这是一个测试合约!"
:其作用是为了输出中文(solidity中不支持直接输出中文)在汉字字符串前加上
unicode
。
MyContract1.js
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| const { ethers } = require("hardhat");
describe("MyContract", function () { it("Here is demo", async function () {
const myContract = await ethers.getContractFactory("MyContract1"); const con = await myContract.deploy(); const owner = await con.getOwner();
console.log("MyContract 的拥有者是 " + owner); }); });
|
解读:
describe("MyContract", function (){})
: 创建测试套件,名称为 “MyContract”;
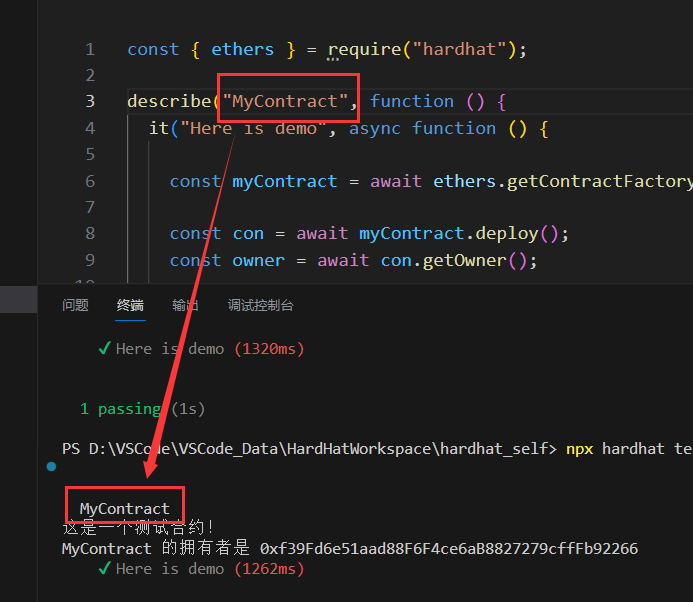
it("Here is demo", async function () {}
:创建测试用例,名称为 “Here is demo”(可以在一个describe
中创建多个it
);
const myContract = await ethers.getContractFactory("MyContract1")
: 获取 “MyContract1” 智能合约的工厂对象;
const con = await myContract.deploy()
: 部署 “MyContract1” 智能合约;
const owner = await con.getOwner()
: 调用智能合约中的 getOwner() 方法获取合约的拥有者。
3.3 测试合约(有参构造器)
MyContract2.sol
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| // SPDX-License-Identifier: UNLICENSED pragma solidity ^0.8.9;
import "hardhat/console.sol";
contract MyContract2 { address owner; string name;
constructor(string memory _name) { owner = msg.sender; name = _name; }
function getOwner() external view returns(address) { return owner; }
function print() external view { console.log(unicode"这是MyContract1合约"); }
function setName(string memory _name) external { name = _name; }
function getName() external view returns(string memory) { return name; } }
|
MyContract2.js
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| const { ethers } = require("hardhat");
describe("MyContract2", async function () {
it("To test ethers.provider.getStorageAt()", async function(){
const MyContract2 = await ethers.getContractFactory("MyContract2");
const contract = await MyContract2.deploy("libiyou"); console.log(contract);
const owner = await contract.getOwner(); console.log(`owner => ${owner}`);
const name1 = await contract.getName(); console.log(`name1 => ${name1}`);
await contract.setName("biyou"); const name2 = await contract.getName(); console.log(`name2 => ${name2}`);
await contract.print();
})
})
|
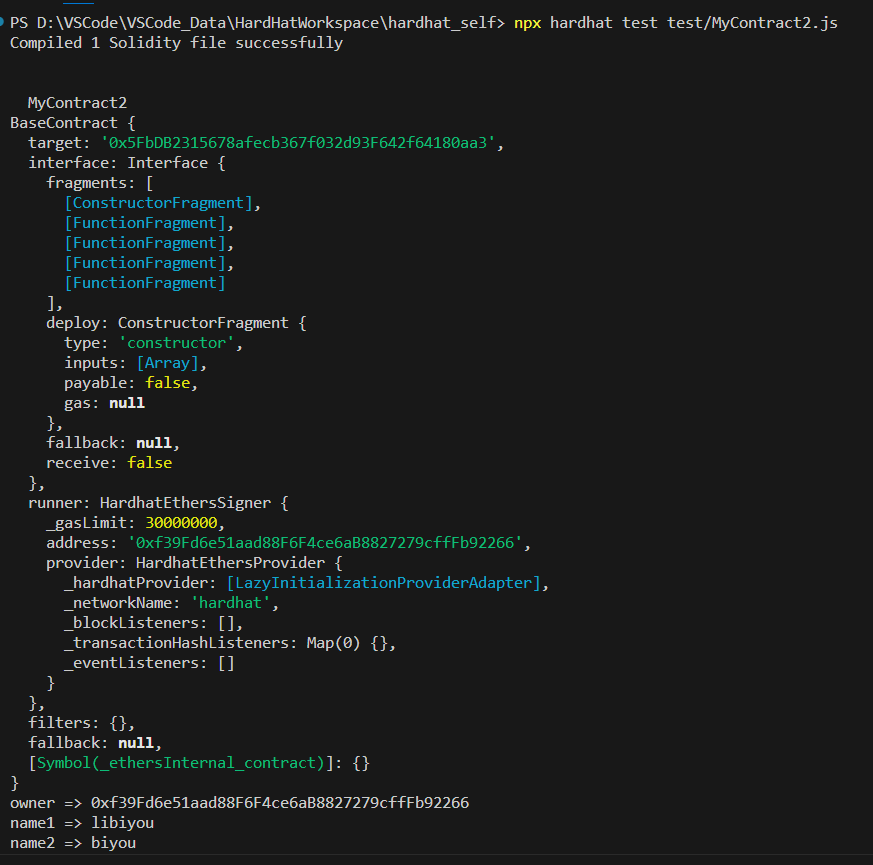
4. 将合约部署到本地开发节点
4.1 语法格式
启动本地节点命令:
npx命令:
1
| npx hardhat run scripts/deploy_MyContract2.js --network localhost
|
js代码:
1 2 3 4 5 6 7 8 9 10 11 12
| const hre = require("hardhat");
async function main() { const toDeployContract = ""; const contract = await hre.ethers.deployContract(toDeployContract,[params...],{ value: }); }
main().catch((error) => { console.error(error); })
|
4.2 部署合约 (无参构造器)
MyContract1.sol
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| // SPDX-License-Identifier: UNLICENSED pragma solidity ^0.8.9;
import "hardhat/console.sol";
contract MyContract1 { address owner; string name;
constructor() { owner = msg.sender; }
function getOwner() external view returns(address) { return owner; }
function print() external view { console.log(unicode"这是MyContract1合约"); }
function setName(string memory _name) external { name = _name; }
function getName() external view returns(string memory) { return name; } }
|
deploy_MyContract1.js
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| const hre = require("hardhat");
async function main() { const contract = await hre.ethers.deployContract("MyContract1"); await contract.waitForDeployment();
console.log(await contract.getAddress());
await contract.setName("biyou"); let name1 = await contract.getName(); console.log(name1); contract.print(); }
main().catch((error) => { console.error(error); })
|
运行结果
:

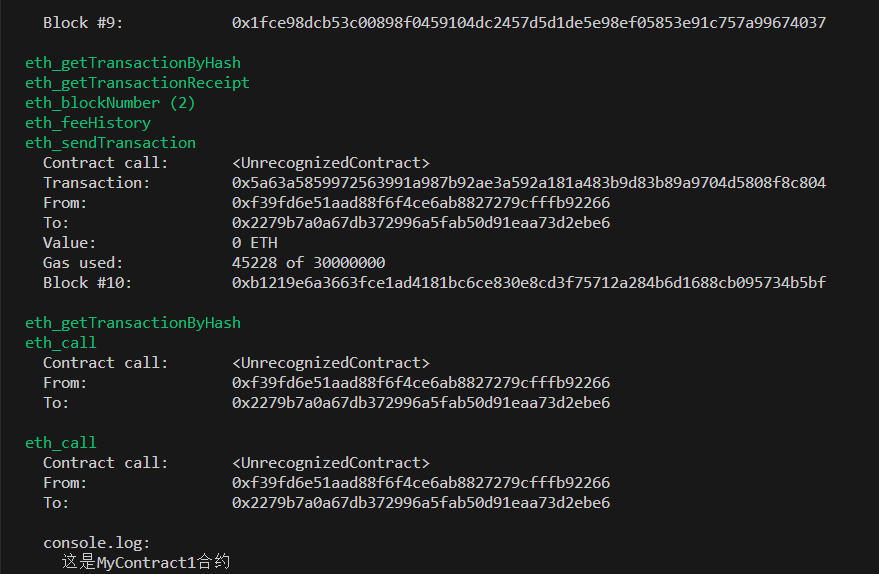
4.3 部署合约 (有参构造器)
MyContract2.sol
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| // SPDX-License-Identifier: UNLICENSED pragma solidity ^0.8.9;
import "hardhat/console.sol";
contract MyContract2 { address owner; string name;
constructor(string memory _name) { owner = msg.sender; name = _name; }
function getOwner() external view returns(address) { return owner; }
function print() external view { console.log(unicode"这是MyContract1合约"); }
function setName(string memory _name) external { name = _name; }
function getName() external view returns(string memory) { return name; } }
|
deploy_MyContract2.js
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| const hre = require("hardhat"); const { ethers } = require("hardhat");
async function main() { const contract = await hre.ethers.deployContract("MyContract2",["lby"]); const address = await contract.getAddress(); console.log(address); let slot0 = await ethers.provider.getStorage(address, 0); let slot1 = await ethers.provider.getStorage(address, 1); console.log(`slot0 => ${slot0}\nslot1 => ${slot1}`);
await contract.setName("biyou"); let name = await contract.getName(); console.log(`name => ${name}`);
slot0 = await ethers.provider.getStorage(address, 0); slot1 = await ethers.provider.getStorage(address, 1); console.log(`slot0 => ${slot0}\nslot1 => ${slot1}`); }
main().catch((error)=>{ console.log(error); })
|
运行结果
:

5. 将合约部署到测试网络
这里以polygon
为例
polygon faucet链接
polygonscan链接
视频教学
5.1 先启动 hardhat
本地开发节点
命令:
5.2 编写配置文件
*hardhat.config.js
*:
1 2 3 4 5 6 7 8 9 10 11 12
| networks: { localhoat: { url: "http://127.0.0.1:8545", chainId: 31337, accounts: [process.env.PRIVATE_KEY] }, polygon: { url: "'https://matic-mumbai.chainstacklabs.com", chainId: 80001, accounts: [POLYGON_PRIVATE_KEY] } }
|
5.3 将本地节点导入小狐狸钱包
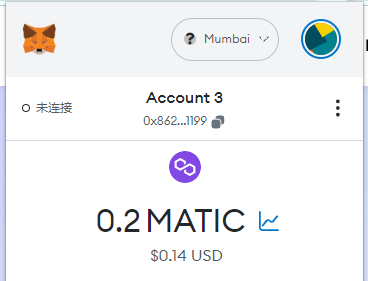
5.4 到 polygon
水龙头领取测试币
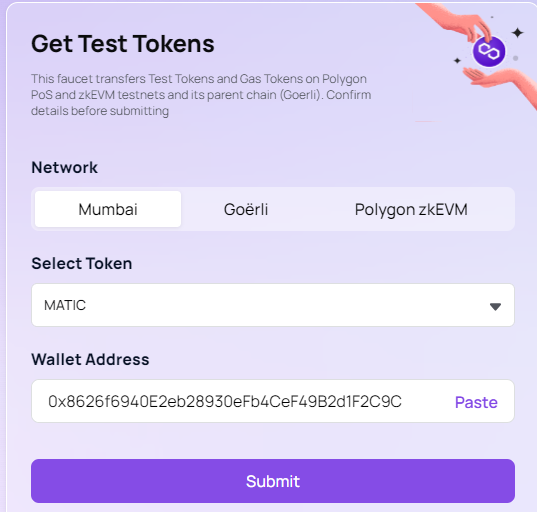
5.将合约部署到polygon
测试网
执行如下命令
1
| npx hardhat run scripts/deploy_MyContract2.js --network polygon
|

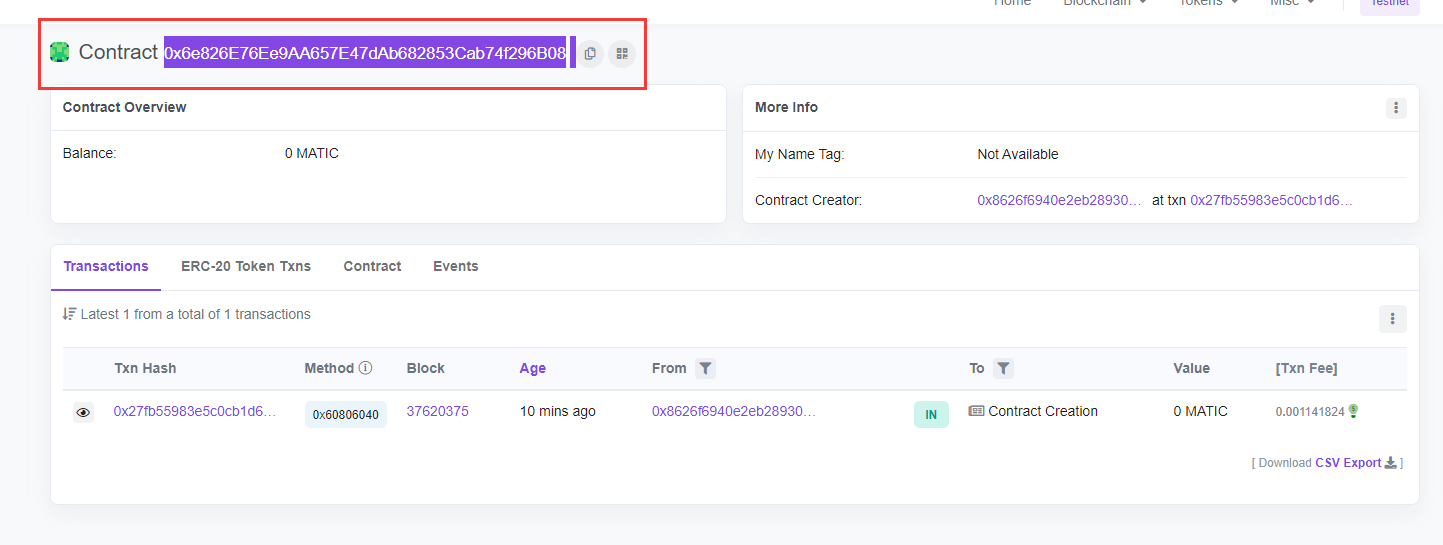